Joint control Tutorial: The Pose Init¶
On this page
NAOqi Motion - Overview | API | Tutorial
Introduction¶
This tutorial explains how to use joint control API in the context of the SoftBank Robotics
definition of the “NAO initial position” known as Stand Init.
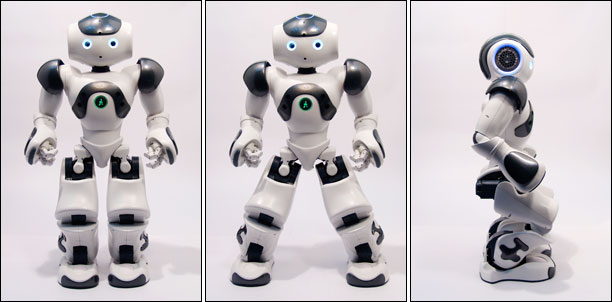
Download¶
Please refer to the section: Python SDK - Installation Guide for any troubleshooting linked to python.
#! /usr/bin/env python
# -*- encoding: UTF-8 -*-
"""Example: PoseInit - Small example to make Nao go to an initial position."""
import qi
import argparse
import sys
def main(session):
"""
PoseInit: Small example to make Nao go to an initial position.
"""
# Get the services ALMotion & ALRobotPosture.
motion_service = session.service("ALMotion")
posture_service = session.service("ALRobotPosture")
# Wake up robot
motion_service.wakeUp()
# Send robot to Stand Init
posture_service.goToPosture("StandInit", 0.5)
# Go to rest position
motion_service.rest()
if __name__ == "__main__":
parser = argparse.ArgumentParser()
parser.add_argument("--ip", type=str, default="127.0.0.1",
help="Robot IP address. On robot or Local Naoqi: use '127.0.0.1'.")
parser.add_argument("--port", type=int, default=9559,
help="Naoqi port number")
args = parser.parse_args()
session = qi.Session()
try:
session.connect("tcp://" + args.ip + ":" + str(args.port))
except RuntimeError:
print ("Can't connect to Naoqi at ip \"" + args.ip + "\" on port " + str(args.port) +".\n"
"Please check your script arguments. Run with -h option for help.")
sys.exit(1)
main(session)